How to use apex.server.process in async mode?
I saw this first time in Vincent’s blog. This is References from Vincent Morneau Blog
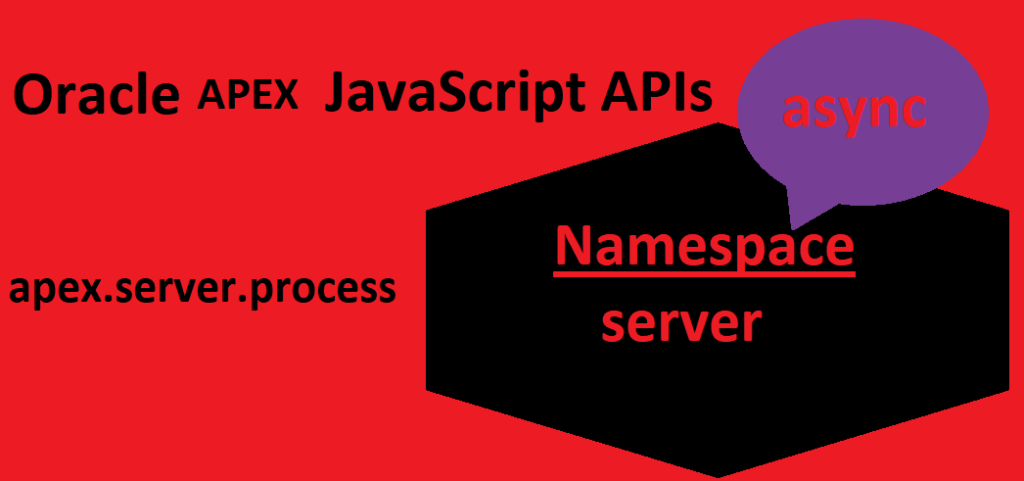
apex.server.process namespace works in async mode, meaning whenever you hit the process it never waits to complete.
Let’s take below example.
- AJAX process name : GET_EMP_DATA
- Description: This process bring data from ORDS REST API.
function get_data(p_url, p_pegination) {
return apex.server.process('GET_EMP_DATA', {
pageItems: "#P28_NEW",
x01: p_pegination,
x02: p_url
}, {
dataType: 'text',
done: function (pData) {
console.log(pData);
alert('pData');
$('#EMP_DATA').append(pData);
}
}, {
loadingIndicator: "#EMP_DATA",
loadingIndicatorPosition: "append"
});
}
To run the above AJAX function we create one function like below
function run() {
console.log('Start');
var result = get_data('https://apex.oracle.com/pls/apex/doctor_app/data/getemp/', '2');
console.log(result);
console.log('End');
}
When you run the run() function it never waits for get_data to complete.
To achieve this we need to use async / await approach. Let’s try below function
async function run() {
console.log('Start');
var result = await get_data('https://apex.oracle.com/pls/apex/doctor_app/data/getemp/', '2');
console.log(result);
console.log('End');
}
Now when we run the run() function it will wait for get_data to complete and return into result.
To check your data using below json parsing query.
SELECT dt.*
FROM dual,
json_table (apex_web_service.make_rest_request(
p_url => 'https://apex.oracle.com/pls/apex/doctor_app/data/getemp/',
p_http_method => 'GET'),
'$.items[*]' COLUMNS ( empno number path '$.empno' ,
ename VARCHAR2(200) path '$.ename' ,
job VARCHAR2(200) path '$.job' ,
mgr number path '$.mgr' ,
hiredate date path '$.hiredate' ,
salary number path '$.sal' ,
comm number path '$.comm' ,
deptno number path '$.deptno'
))dt;
.done promise
apex.server.process(
'PROCESS_NAME',
{f01: '<ARRAY>',
x01: '',
pageItems: '#<PAGE_ITEMS>'},
{
dataType: "text" // 'json'
}
).done(function(data){
// do something
}
apex.region("region_id").refresh();
});