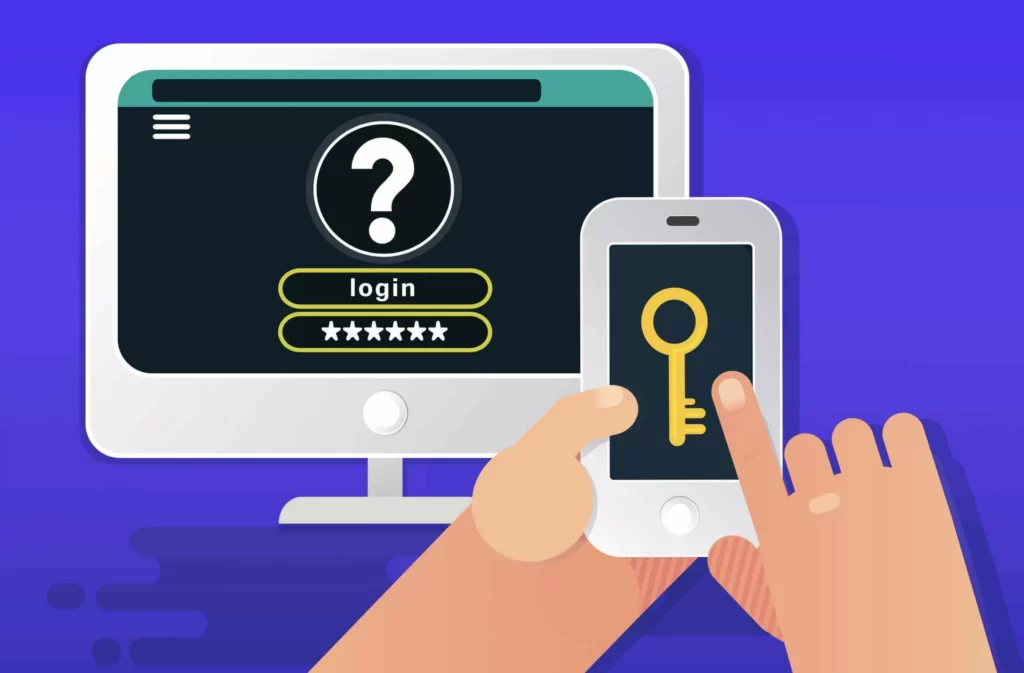
Custom Authentication is the Authentication type where we can use the custom logic to login into the APEX application. They need to be written in PLSQL and must return a Boolean value.
An “Authentication Function Name” is required to define under the Application authentication settings.
Steps to define the Boolean function
- Go to Shared components
- Authentication Schemes
- Create scheme
- Select Scheme Type as Custom
- Define the Boolean function
Below are screenshots
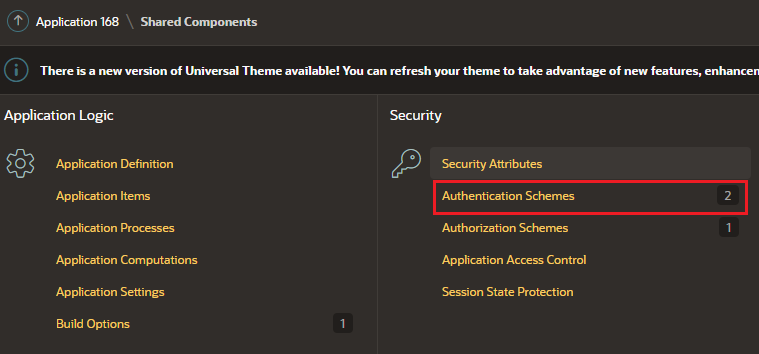
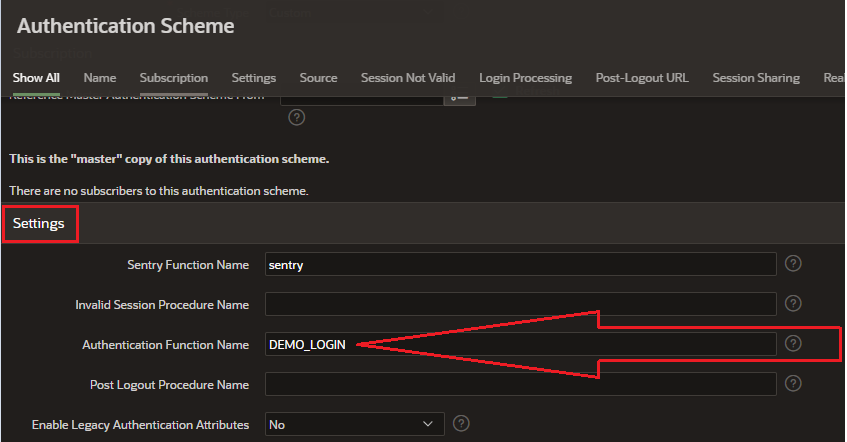
Custom Function sample code.
CREATE OR REPLACE FUNCTION demo_login (p_username IN VARCHAR2,
p_password IN VARCHAR2)
RETURN BOOLEAN
AS
BEGIN
IF UPPER (p_username) = 'DEMO' AND UPPER (p_password) = 'DEMO'
THEN
RETURN TRUE;
ELSE
RETURN FALSE;
END IF;
END;
Another sample code with table example from Help text
FUNCTION my_authentication (p_username IN VARCHAR2, p_password IN VARCHAR2)
RETURN BOOLEAN
IS
l_user my_users.user_name%TYPE := UPPER (p_username);
l_id my_users.id%TYPE;
l_hash my_users.password_hash%TYPE;
BEGIN
BEGIN
SELECT id, password_hash
INTO l_id, l_hash
FROM my_users
WHERE user_name = l_user;
EXCEPTION
WHEN NO_DATA_FOUND
THEN
l_hash := '-invalid-';
END;
RETURN l_hash =
RAWTOHEX (
sys.DBMS_CRYPTO.hash (
sys.UTL_RAW.cast_to_raw (p_password || l_id || l_user),
sys.DBMS_CRYPTO.hash_sh512));
END;
Places to put your Boolean function code.
- In the Database
- Source text area in Authentication
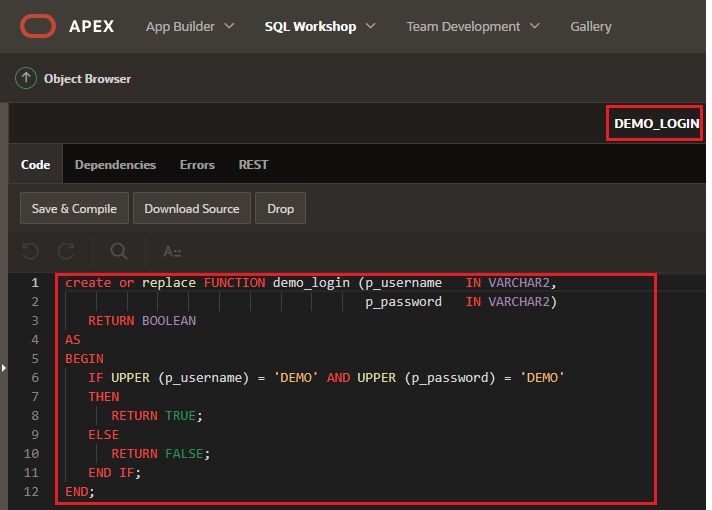
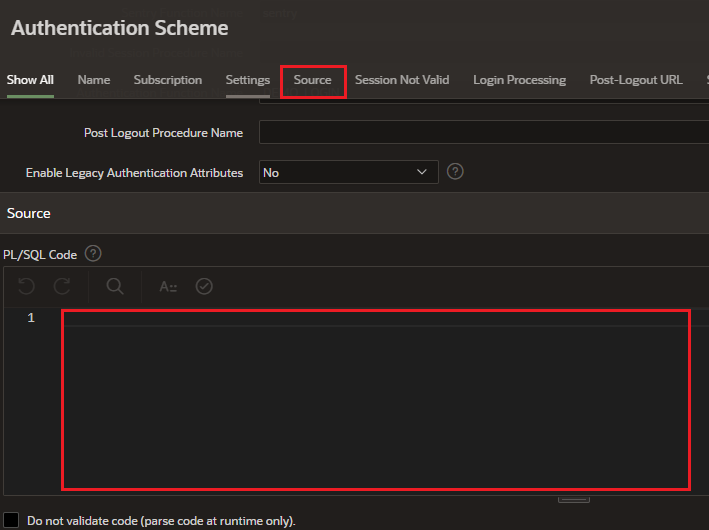
Application Login Page setup
If you have used the function parameters names like p_username and p_password, then you don’t need to make any changes. by default, APEX_AUTHENTICATION.login function has the same parameter name. If your Boolean function parameters are not the same, it the time to correct them.
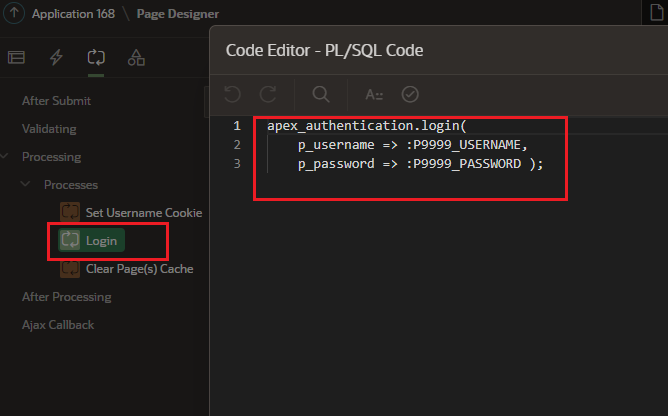
We are all set to use the custom login, Run the application and put in your credentials.
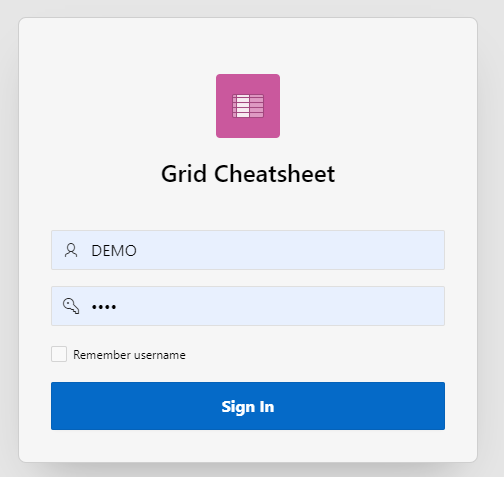
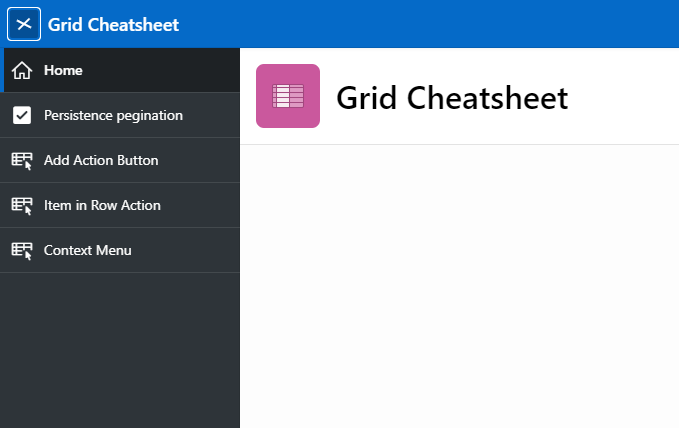