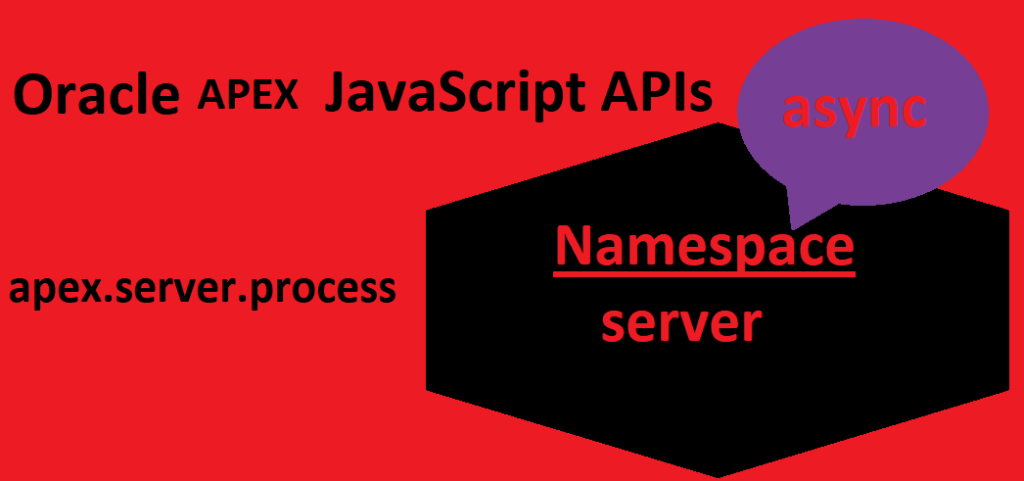
apex.server.process namespace works in async mode, meaning whenever you hit the process it never waits to complete.
Let’s take below example.
AJAX process name : GET_EMP_DATA
Description: This process bring data from ORDS REST API.
function get_data(p_url, p_pegination) {
return apex.server.process('GET_EMP_DATA', {
pageItems: "#P28_NEW",
x01: p_pegination,
x02: p_url
}, {
dataType: 'text',
done: function (pData) {
console.log(pData);
alert('pData');
$('#EMP_DATA').append(pData);
}
}, {
loadingIndicator: "#EMP_DATA",
loadingIndicatorPosition: "append"
});
}
To run the above AJAX function we create one function like below
function run() {
console.log('Start');
var result = get_data('https://apex.oracle.com/pls/apex/ashish_portfolio/hr/test_emp', '2');
console.log(result);
console.log('End');
}
When you run the run() function it never waits for get_data to complete.
To achieve this we need to use async / await approach. Let’s try below function
async function run() {
console.log('Start');
var result = await get_data('https://apex.oracle.com/pls/apex/ashish_portfolio/hr/test_emp', '2');
console.log(result);
console.log('End');
}
Now when we run the run() function it will wait for get_data to complete and return into result.