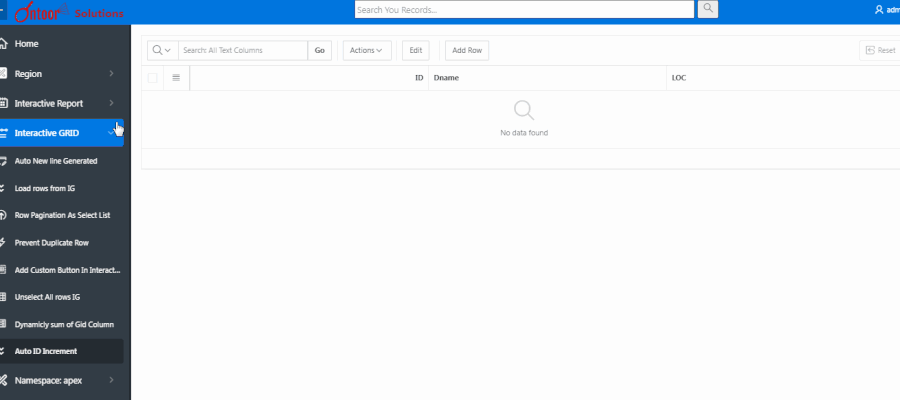
Oracle APEX Interactive Grids offer a powerful and flexible way to interact with data. A common requirement is to have auto-incrementing line numbers for each row, particularly when adding new rows. This article explores how to achieve this feature in Oracle APEX using dynamic actions and JavaScript.
Overview
To add auto-incrementing line numbers in an Interactive Grid, you can use a global JavaScript variable to maintain the row count. Each time a new row is added, the row number is incremented, providing a consistent numbering system.
Solution 1: Incremental Line Numbers
In this solution, you increment the line number each time a row is initialized in the Interactive Grid.
Step 1: Declare a Global JavaScript Variable
To start, declare a global JavaScript variable to store the row number. This variable is used to dynamically increment the row number as new rows are added.
- Open Page Designer: Navigate to the Oracle APEX page where your Interactive Grid is located.
- Declare the Global Variable: In the “JavaScript” section, add the following code to the “Function and Global Variable Declaration”:
var i = 0; // Global variable to maintain the row count
Step 2: Create a Dynamic Action
Create a dynamic action to update the row number in the “ID” column when a new row is added to the Interactive Grid.
- Event: Set the event to “Custom.”
- Custom Event: Enter “row-initialization[Interactive Grid].”
- Selection Type: Choose “Column.”
- Columns: Select the “ID” column, or the column where the line number should be displayed.
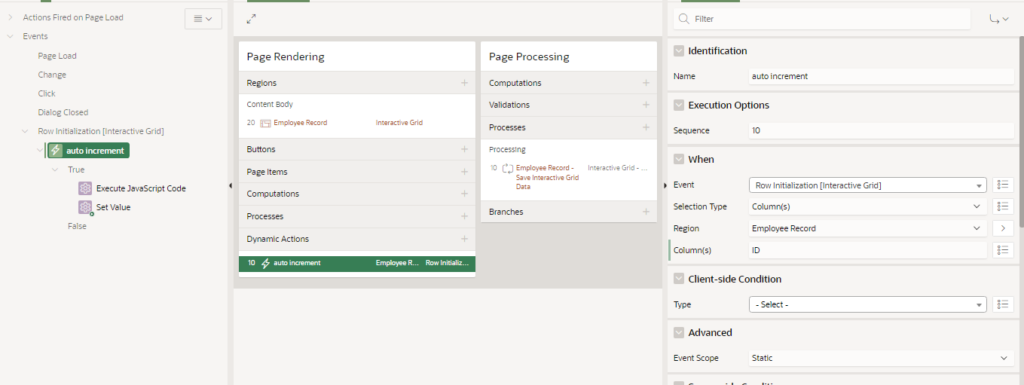
Action 1: Execute JavaScript Code
This action increments the row number every time the Interactive Grid initializes a new row.
- Action Type: “Execute JavaScript Code.”
- JavaScript Code:
i += 1; // Increment the row count
Action 2: Set Value
This action sets the “ID” column to the current value of the global variable.
- Set Type: “JavaScript Expression.”
- JavaScript Expression:
i
- Affected Elements: Select the “ID” column.
i
Additional Considerations
- Read-Only Column: Ensure the “ID” column is set to “Read-only – always” to prevent manual changes to the row number.
Solution 2: Set Line Numbers Based on Total Records
In this solution, you use the total number of records in the Interactive Grid to set the initial row number. This approach is useful if you need a consistent row numbering even when rows are removed or reordered.
Step 1: Create a Dynamic Action
Create a dynamic action to set the row number based on the total number of records.
- Event: Set the event to “Custom.”
- Custom Event: “row-initialization[Interactive Grid].”
- Selection Type: “Column.”
- Columns: Select the “ID” column.
Action 1: Execute JavaScript Code
This action sets the global variable to the total number of records in the Interactive Grid.
- Action Type: “Execute JavaScript Code.”
- JavaScript Code:
i = apex.region("my_ig").widget().interactiveGrid("getViews", "grid").model.getTotalRecords(); // Get the total number of records
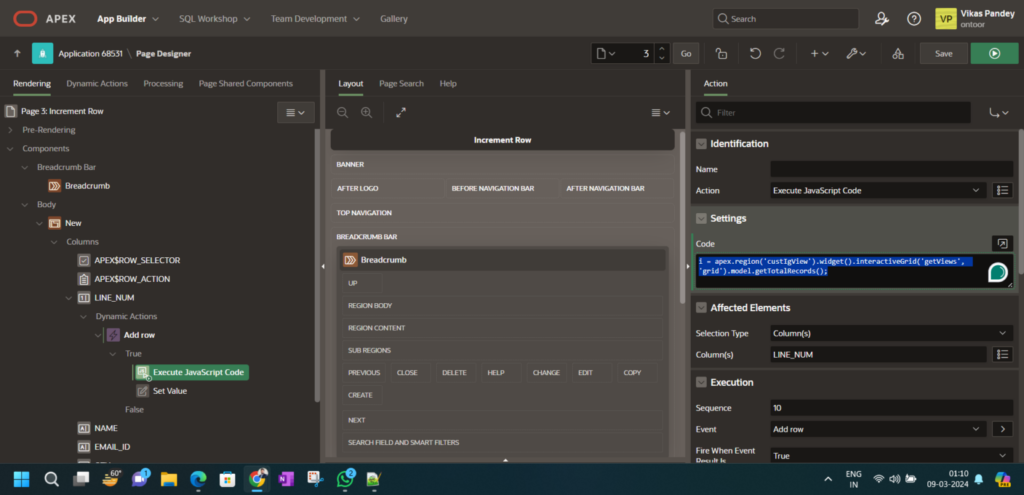
Action 2: Set Value
This action sets the “ID” column to the current value of the global variable.
- Set Type: “JavaScript Expression.”
- JavaScript Expression:
i
- Affected Elements: Select the “ID” column.
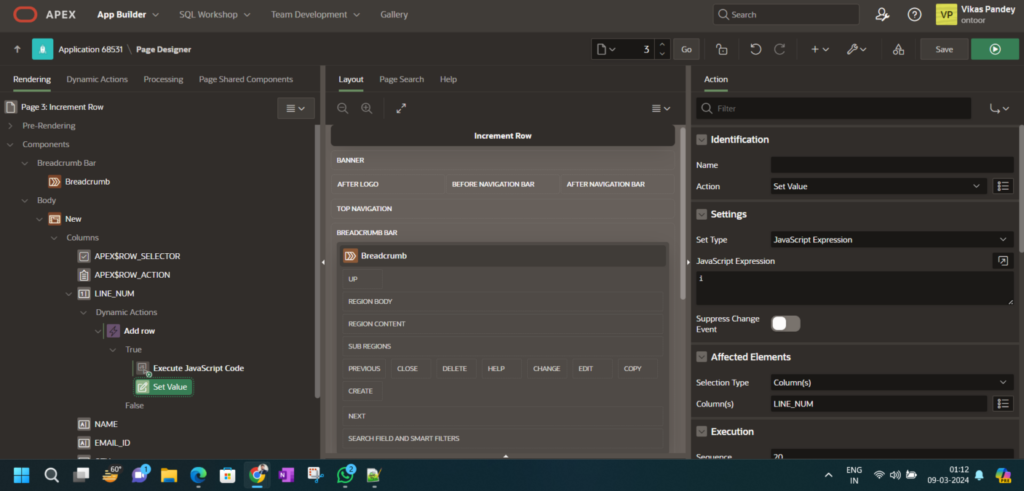
Additional Considerations
- Read-Only Column: To prevent manual edits to the row number, ensure the “ID” column is set to “Read-only – always.”
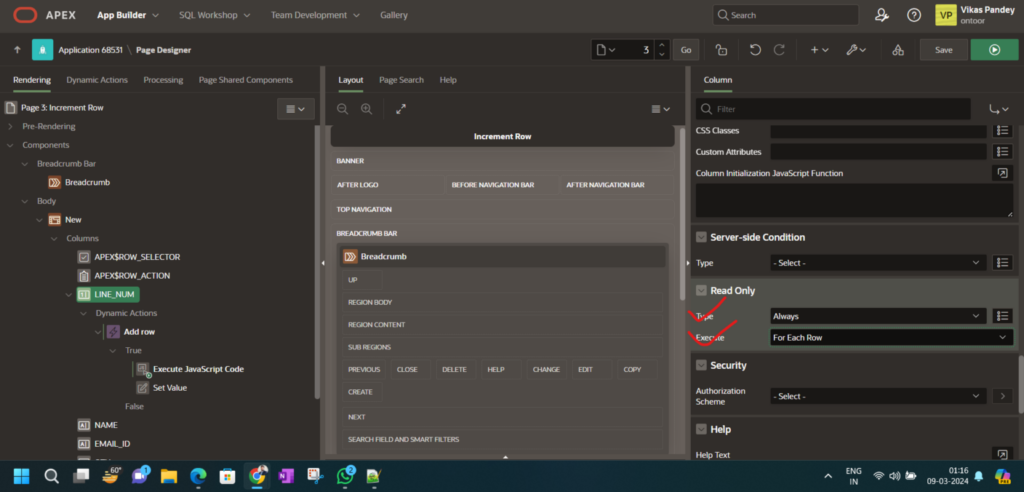
Conclusion
Adding auto-incrementing line numbers in Oracle APEX Interactive Grid can be achieved using global JavaScript variables and dynamic actions. Solution 1 offers a simple incremental approach, while Solution 2 calculates the initial row number based on the total records in the grid. Both methods provide a reliable way to maintain consistent line numbers when new rows are added. If you encounter issues, ensure the JavaScript code is correct and that the appropriate dynamic actions are configured.