In this article, we’ll guide you through adding dynamic tooltips to Interactive Grid (IG) headers in Oracle APEX, utilizing a lookup table for flexibility and customization. This feature improves user interaction by providing context-sensitive information directly within the grid headers
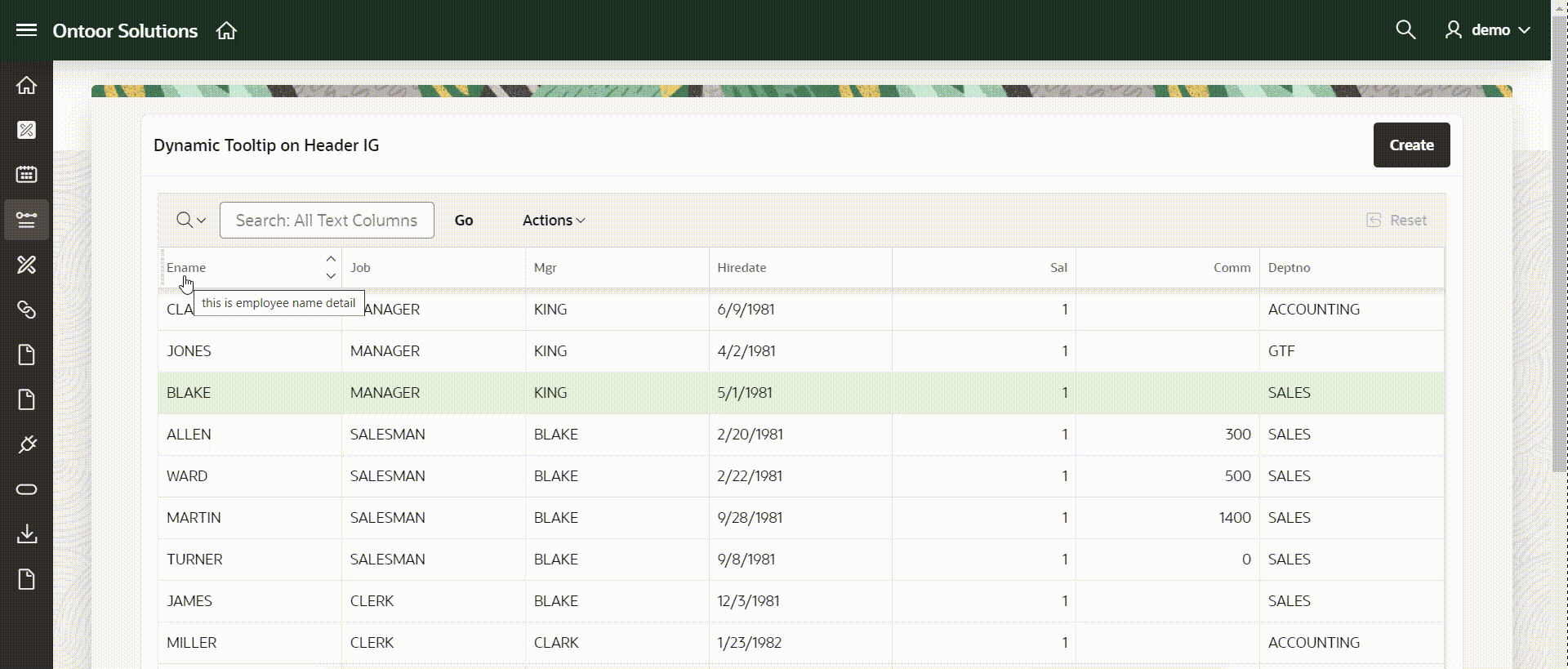
Overview
The goal is to dynamically display tooltips for column headers in an Oracle APEX Interactive Grid. This approach enhances the grid’s usability by providing users with contextual hints and descriptions directly within the grid headers. Tooltips can be particularly useful for clarifying column contents and guiding user interaction, making your application more intuitive and user-friendly.
Step-by-Step Implementation
1. Create the Lookup Table
Start by creating a table to store tooltip descriptions. This table will link the tooltip text to the respective column headers using column identifiers. Here’s how you can set it up:
SQL to Create the Lookup Table:
CREATE TABLE "LOOKUPTABLE"
(
"LOOKUP_ID" NUMBER PRIMARY KEY,
"LOOKUP_DESC" VARCHAR2(255),
"LOOKUP_CODE" VARCHAR2(100) -- This should match the column names in the IG
);
- LOOKUP_DESC: The description or tooltip text.
- LOOKUP_CODE: The code that links to column headers in the Interactive Grid.
2. Populate the Lookup Table
Create a form or manually insert data into the LOOKUPTABLE
. Ensure that the LOOKUP_CODE
values correspond to the static IDs of the columns in your Interactive Grid. For instance, if a column in the IG has a static ID, the LOOKUP_CODE tooltip should also be ENAME
.
-- Insert tooltip data into lookup_table
INSERT INTO lookup_table (lookup_id, lookup_desc, lookup_code) VALUES (1, 'Employee Name', 'ENAME');
INSERT INTO lookup_table (lookup_id, lookup_desc, lookup_code) VALUES (2, 'Employee Manager', 'MGR');
INSERT INTO lookup_table (lookup_id, lookup_desc, lookup_code) VALUES (3, 'Hire Date', 'HIREDATE');
-- Add more entries as needed
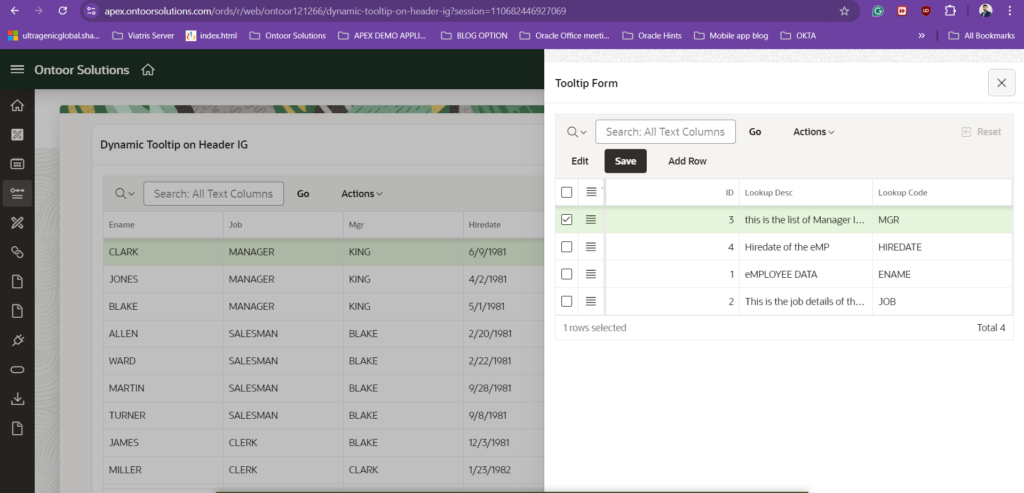
3. Create a shortcut in Function Boolean returning for Tooltip Data
Write a PL/SQL function to fetch tooltip data from the lookup table and return it in JSON format. JavaScript will use this JSON data to apply tooltips.
PL/SQL Function Code:
DECLARE
l_data CLOB; -- Using CLOB for large JSON data
BEGIN
-- Generate JSON array from lookup table
SELECT JSON_ARRAYAGG(
JSON_OBJECT(
'TooltipID' VALUE LOOKUP_CODE || '_HDR',
'TooltipDesc' VALUE LOOKUP_DESC
)
) INTO l_data
FROM LookupTable
WHERE LOOKUP_CODE IS NOT NULL;
RETURN l_data; -- Return JSON array of tooltips
END;
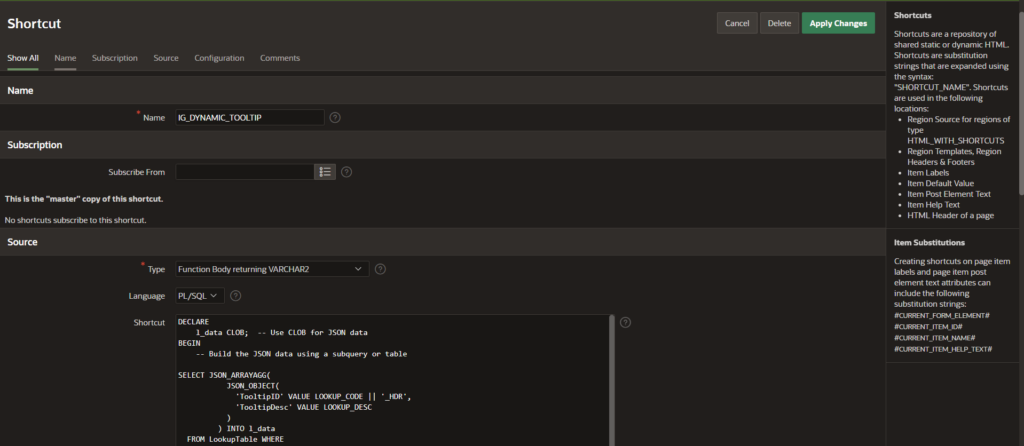
This function aggregates tooltip information into a JSON array, where each object includes the column identifier and description.
4. Implement JavaScript for Dynamic Tooltips
Integrate JavaScript on the function global variable declaration containing the Interactive Grid to apply tooltips dynamically based on the JSON data returned by the PL/SQL function.
JavaScript Code:
(function($){
$(function(){
var regionStaticId = "my_emp_id"; // Replace with your Interactive Grid's static ID
setIgHeaderHelp(regionStaticId).on("interactivegridviewchange interactivegridreportsettingschange", function(event, ui) {
setIgHeaderHelp(this.id);
});
});
function setIgHeaderHelp(region){
var region$ = $($x(region));
// Fetch tooltip data using the defined shortcut
$.each(IG_DYNAMIC_TOOLTIP, function(i, tooltipData){
region$.find([$x(tooltipData.TooltipID)]).parent("th").attr("title", tooltipData.TooltipDesc);
});
return region$;
}
})(apex.jQuery);
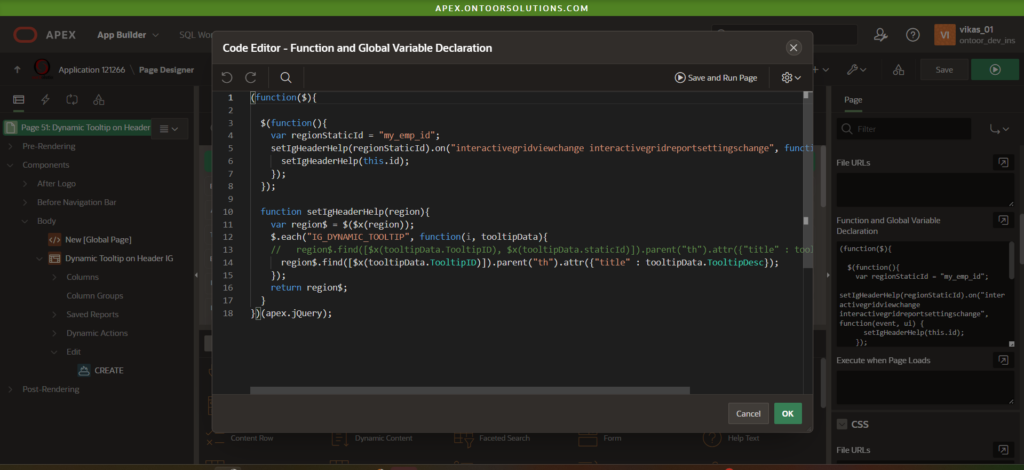
Explanation of the JavaScript Code:
- Initialization: The script runs when the document is ready, ensuring the Interactive Grid is fully loaded.
- Applying Tooltips: The
setIgHeaderHelp
function updates the column headers with tooltip text based on the JSON data. It targets the correct headers using the IDs and sets theirtitle
attributes to display the tooltips. - Dynamic Updates: The tooltips are re-applied when the grid view or settings change, maintaining up-to-date and relevant information.
- Event Handling: The
setIgHeaderHelp
function is called initially and then again whenever the Interactive Grid view or report settings change, ensuring tooltips are updated dynamically. - Tooltip Application: The
setIgHeaderHelp
function fetches the tooltip data and sets it on the column headers. It uses jQuery to find elements based on the static IDs and applies thetitle
attribute with the corresponding tooltip description.
Conclusion
By implementing dynamic tooltips for Interactive Grid headers, you enhance the usability and accessibility of your Oracle APEX application. This approach allows for easy updates and maintenance of tooltip content, providing users with relevant information directly within the grid interface.
Stay tuned to our blog for further enhancements and best practices in Oracle APEX. For any questions or need additional support, feel free to reach out!
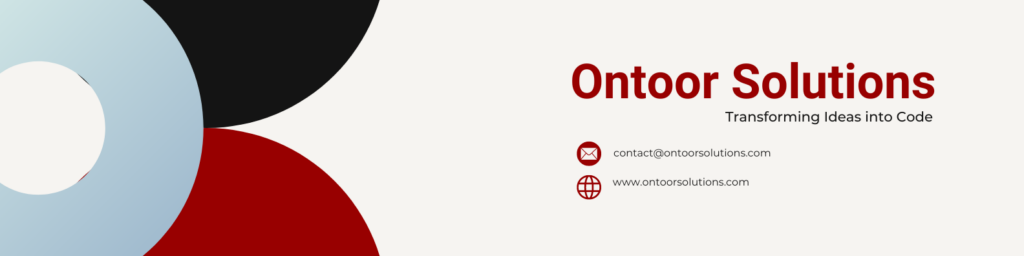