In Oracle APEX, passing values from one item to another using JavaScript can be accomplished in a few steps. Below is an example that demonstrates how to do this. Let’s assume you have two page items: P3_EMAIL
and P3_EMAILVIEW
. You want to pass the value from
to P3_EMAIL
P3_EMAILVIEW
using JavaScript.
Disable item P3_EMAILVIEW using the disabled property so that no one can enter manually.
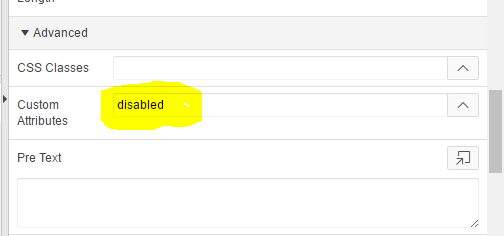
- Create a Dynamic Action:
- Go to the Page Designer.
- Select
Button.Show_BTN
- Under the “Dynamic Actions” section, create a new Dynamic Action.
- Set the “Name” to something like “Set
Value”.P3_EMAILVIEW
- Set the “Event” to
Click
(or another event as per your requirement). - Set “Selection Type” to
Button
and choose
.Show_BTN
- Add a True Action:
- Under “True Actions,” add action.
- Select “Action” as
Execute JavaScript Code
. - In the “Code” section, add the JavaScript code
- This code uses the APEX JavaScript API:
apex.item('
to get the value ofP3_EMAIL
').getValue()
.P3_EMAIL
apex.item('
to set the value ofP3_EMAILVIEW
').setValue(item1Value)
.P3_EMAILVIEW
- Save and Run:
- Save your changes.
- Run the page to test the functionality.
Affected Element > Selection Type - Items> items- P3_EMAILVIEW
var item1Value = apex.item('P3_EMAIL').getValue(); // Get the value of P3_EMAIL
apex.item( "P3_EMAILVIEW" ).setValue( item1Value);
//If you want to suppress events on this value change
apex.item( "P3_EMAILVIEW" ).setValue( item1Value, "", true );
Now, whenever the value of
changes, the value of P3_EMAIL
will be automatically updated with the same value.P3_EMAILVIEW
For POPUP Lovs
apex.item( "P1_ITEM" ).setValue( "10", "SALES", true );
// first Param "10" is return value to the LOV
// Second Param "SALES" is display value to the LOV
// Third Param is to suppress events if any on the taget item
Additional Notes:
- Validation: Ensure that the items (
andP3_EMAIL
) are on the same page, or adjust the item names accordingly if they are on different pages.P3_EMAILVIEW
- Customization: Before setting the value, you can customize the JavaScript code to perform additional operations or validations.
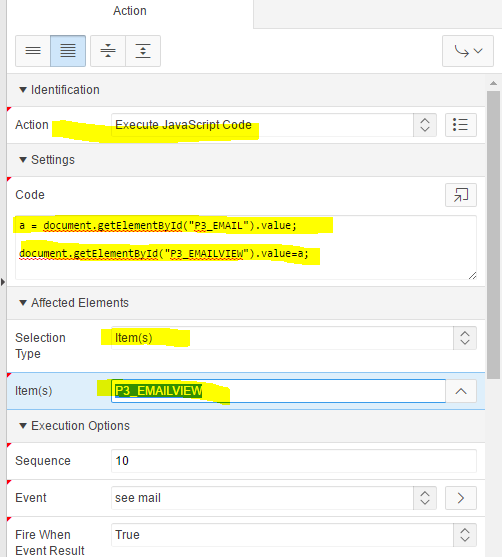
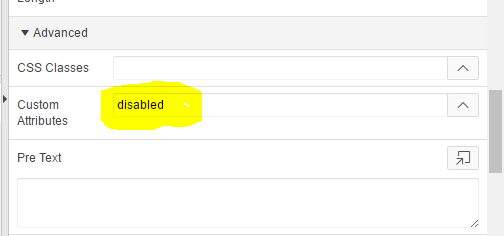
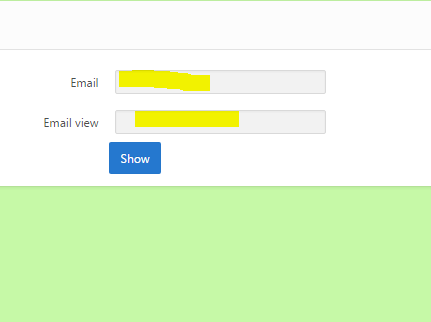
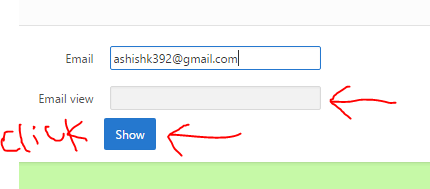
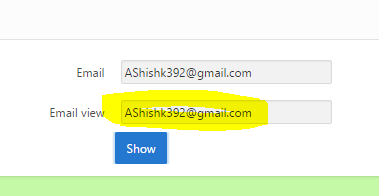