APEX_ITEM is a built-in API that provides many types of input types using SQL queries. We use it for Interactive reports and classic reports. This article is about the checkboxes in APEX reports. Let’s explore the checkbox use case and try some customization.
Below is the standard query that adds the checkbox to the report
SELECT APEX_ITEM.checkbox2 (p_idx => 1,
p_value => empno,
p_attributes => ' class="checkbox"')
"SELECT",
'<span><i class="fa fa-edit"></i></span>' edit,
empno,
ename,
job,
mgr,
hiredate,
sal,
comm,
deptno,
end_date
FROM emp
In the above query the First column (Select), checkbox2 function has many parameters. Here I am using three of them p_idx id for the input, p_value to assign value to the item /input, and p_attributes is adding other attributes like class. We can add other things using p_attributes including styles, data attributes etc.
The second column is to edit a single row, Add one more Page Button to take the multi checkboxes and take them for bulk operations.
Few more steps are to get the checkbox and edit icon after putting the query.
- Click on “Select” and “Edit” Column
- Go to Column Attributes
- Scroll to the Security section
- Uncheck “Escape special characters“
The above steps only provide checkboxes in report rows, for the column header add the below input tag. Input class will be used to trigger the event on click of this check.
<input class="checkall" type="checkbox">
Check all checkboxes on the header checkbox on click
An additional Javascript function is used to check all the checkboxes in the report. This function needs the report Static ID.
function check_all(p_region_id) {
$('#' + p_region_id + ' th span input.checkall').click(function() {
$('#' + p_region_id + ' td input:checkbox').prop('checked', this.checked);
})
}
- Line number 1 is just the function name and parameter.
- Line number 2 is to catch the click event on the header checkbox click.
- Line number 3 is to check and uncheck rows.
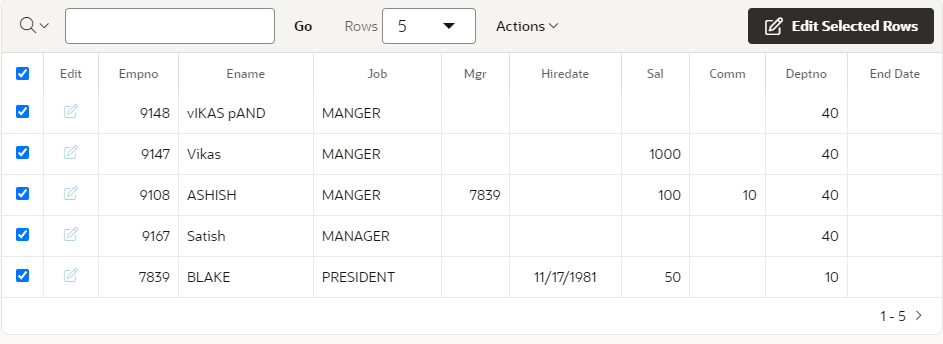
Enable disable Single edit button and Multi-Edit Button
I have created a new function disable_enable_edit that needs region Static ID similar to the above functions.
- Line Numner1: Function name parameter
- Line Number 2: Calculates the count of checked rows.
- Line Number 3: Calculates the count of all checkboxes
- Line Number 4: Condition if a single checkbox is selected
- Line Number 8: Else condition if multiple checkboxes are checked
- Line Number 5, 6, 9, 10: These four lines are used to disable and enable the row edit button and Page Button conditionally
- After Line Number 13: If the count of all checked rows is equal to the total number of checkboxes, it check checks the header checkbox else uncheck it.
function disable_enable_edit(p_region_id) {
var check_count = parseInt($('#' + p_region_id + ' td input:checkbox:checked').length);
var all_checkboxCount = parseInt($('#' + p_region_id + ' td input:checkbox').length);
if (check_count > 1) {
$('#' + p_region_id + ' tbody tr td span i.fa-edit').parent('span').parent('a').addClass('apex_disabled');
$('.edit_btn').addClass('t-Button--hot').removeClass('apex_disabled');
} else {
$('#' + p_region_id + ' tbody tr td span i.fa-edit').parent('span').parent('a').removeClass('apex_disabled');
$('.edit_btn').removeClass('t-Button--hot').addClass('apex_disabled');
}
// Header selection
if (check_count == all_checkboxCount) {
$('#' + p_region_id + ' th span input.checkall').prop('checked', 'checked');
} else
$('#' + p_region_id + ' th span input.checkall').prop('checked', '');
}
Here I am adding/removing the apex_disabled class to the Page button if the number of selections is more than one.
If you want Focus on Edit Button try the below snippet
var check_count = parseInt($('input:checkbox:checked').length);
if ( check_count > 1 ){
// set focus code
$('.edit_btn').focus();
}
Execute all functions using a wrapper.
function execute(p_region_id){
check_all(p_region_id);
disable_enable_edit(p_region_id);
refresh_events(p_region_id);
}
Call the “execute” function on the page load.
Refresh events on Partial refresh
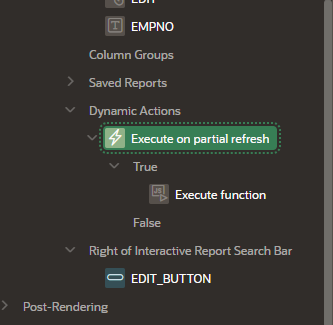
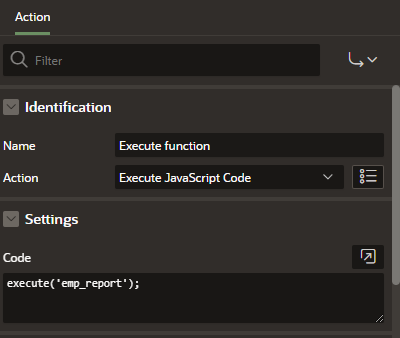
// Refresh events
function refresh_events(p_region_id) {
$('#' + p_region_id + ' td input:checkbox').click(function() {
// call row selection function
disable_enable_edit(p_region_id);
});
$('#' + p_region_id + ' th span input.checkall').click(function() {
// call row selection function
disable_enable_edit(p_region_id);
});
}
To refresh events on Partial refresh, we need to create Dynamic action in Report refresh and execute the above javascript function “refresh_events”
* Right Click on Report
* Click on "Create Dynamic Action"
* Select "After Refresh" as event
* Selection type "Region" and select report
* Add true action as "Execute Javascript code"
* execute('emp_report'); // emp_report is report static ID
Change the Mouse pointer using CSS
.checkall, .checkbox{
cursor: pointer;
}
* checkall is class of report column header checkbox class
* checkbox is class of all checkboxes of data rows