In Oracle APEX applications, it’s often necessary to restrict user input to numeric values, especially in Interactive Grids or other data-entry fields. This article demonstrates how to enforce numeric-only input using jQuery and CSS classes in Oracle APEX, with step-by-step guidance.
JavaScript Code to Restrict Input
The following code snippet binds the keypress
event to elements with a specific CSS class (numeric_cls
), allowing only numeric input (digits 0-9) and blocking non-numeric characters.
$(".numeric_cls").on("keypress", function (e) {
var keyCode = e.which || e.keyCode; // Get the key code from the event
// Allow only numeric characters (ASCII 48-57)
if (keyCode < 48 || keyCode > 57) {
e.preventDefault(); // Block non-numeric input
}
});
$(".numeric_cls").bind("keypress", function (e) {
var keyCode = e.which ? e.which : e.keyCode; // Determine the key code from the event
// Allow only digits (0-9) or a single decimal point
if (!(keyCode >= 48 && keyCode <= 57) && !(keyCode == 46)) {
return false; // Block non-numeric input
}
});
How the Code Works
- Key Code Validation: The code snippet checks the ASCII code of the key press event. It only allows input if the key code is between 48 and 57 (which represents the digits 0-9). If not, it prevents the keypress by returning
e.preventDefault()
. - Binding to a CSS Class: By targeting elements with the
numeric_cls
class, you can restrict input to numeric values for specific Oracle APEX components, such as Interactive Grid columns or page items.
Implementing Numeric-Only Input in Oracle APEX
To apply this code snippet and restrict input to numeric values in Oracle APEX, follow these steps:
Step 1: Assign a CSS Class to the Target Field
- Open Oracle APEX: Navigate to the page where you need to restrict input to numeric values.
- Select the Interactive Grid Column or Page Item:
- If working with an Interactive Grid, select the column you want to restrict to numeric input and assign a CSS class in the “Column Attributes” section.
- For other page items (like text fields), assign the CSS class in the “Element” section.
- Assign the CSS Class:
- Use a unique class name like
numeric_cls
to ensure it is easy to target with jQuery.
- Use a unique class name like
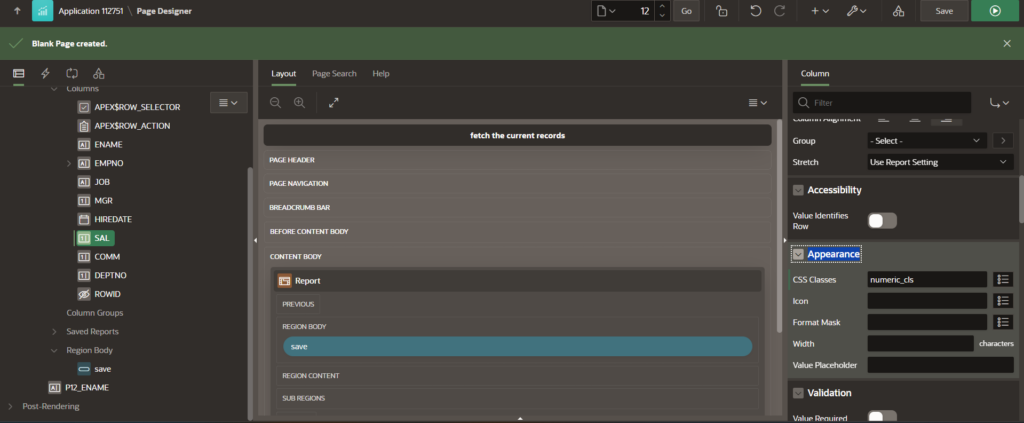
Step 2: Add the jQuery Script
- Include jQuery: Ensure jQuery is included in your Oracle APEX application. If not, add a reference to jQuery in your Execute when page Loads section.
- Add the JavaScript Code:
- In your JavaScript section or script file, insert the code snippet to bind the
keypress
event to elements with thenumeric_cls
class.
- In your JavaScript section or script file, insert the code snippet to bind the
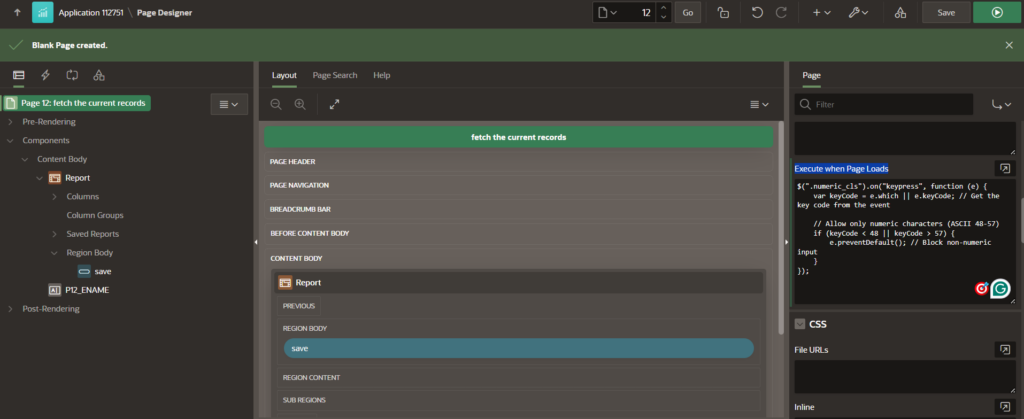
Step 3: Test the Numeric Restriction
- Run the Page: After implementing the code, test your Oracle APEX application to ensure the numeric restriction is working as expected.
- Try Non-Numeric Characters: Attempt to input non-numeric characters to verify they are blocked.
Additional Considerations
- Special Keys: The code snippet doesn’t handle special keys like backspace, delete, or arrow keys. You may need to allow these keys to improve usability.
- Check CSS Class Assignment: Ensure the
numeric_cls
class is assigned to the correct Oracle APEX components.
Conclusion
Restricting Oracle APEX input fields to numeric values is useful for maintaining data integrity and improving user experience. Using jQuery and CSS classes, you can enforce numeric-only input in Interactive Grids and other page items. This guide provides step-by-step instructions for implementing this restriction and highlights additional considerations to ensure proper behavior. If you encounter issues, check for JavaScript errors and confirm the correct CSS class assignment.