Oracle APEX Interactive Grids are powerful tools for managing and interacting with data. A common use case is retrieving a specific value from the current row when a user selects it, enabling you to populate other fields or perform additional processing. This article explains how to retrieve a value from the current row in an Interactive Grid using JavaScript and a dynamic action, with detailed explanations for each step.
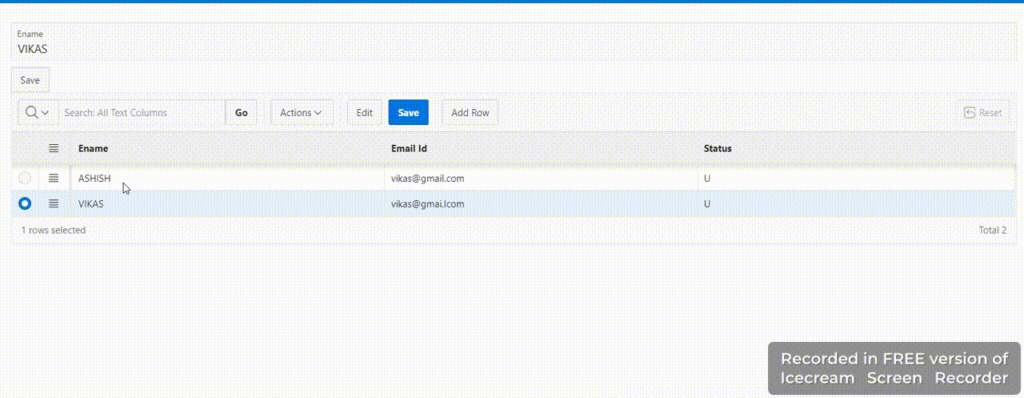
JavaScript Code to Get the Selected Value
The following code snippet retrieves the value from the “ENAME” column of the currently selected row in an Interactive Grid. It then sets this value to another Oracle APEX item, “P5_ENAME,” when the row selection changes.
var full_name; // Variable to store the retrieved value
var model = this.data.model; // Reference to the Interactive Grid's data model
if (this.data && this.data.selectedRecords && this.data.selectedRecords[0]) {
// Get the "ENAME" value from the first selected record
full_name = model.getValue(this.data.selectedRecords[0], "ENAME");
}
// Set the retrieved value to the Oracle APEX item "P5_ENAME"
apex.item("P5_ENAME").setValue(full_name);
Understanding the Code
- Variable Definition:
full_name
is a variable used to store the value retrieved from the Interactive Grid. - Data Model Reference:
model
refers to the Interactive Grid’s data model, allowing access to row data and values. - Check for Valid Data and Selection: The code checks if the data model and selected records are valid, ensuring there is a selected row to retrieve a value from.
- Retrieve the Value: If a row is selected, the code retrieves the “ENAME” value from the selected record using
model.getValue
. - Set the Retrieved Value: After retrieving the value, the code sets it to the Oracle APEX item “P5_ENAME” using
apex.item().setValue
.
Implementing the Dynamic Action
To use the above code to get a value from the current row and set it to another Oracle APEX item, you need to create a dynamic action that triggers when a row is selected in the Interactive Grid. Follow these steps:
- Open Oracle APEX:
- Navigate to the Application Builder and open the page containing your Interactive Grid.
- Create a Dynamic Action:
- Select the Interactive Grid region.
- Go to the “Dynamic Actions” section and create a new dynamic action.
- Set the Event to “Selection Change” to trigger the action when the row selection changes.
- Choose “Region” as the Selection Type and select your Interactive Grid.
- Add the JavaScript Code:
- Within the dynamic action, add an action of type “Execute JavaScript.”
- Paste the JavaScript code snippet to retrieve the selected value from the current row.
- Test the Dynamic Action:
- After applying the code, run the page and select a row in the Interactive Grid.
- Check if the “ENAME” value is set in the “P5_ENAME” item when the row is selected.
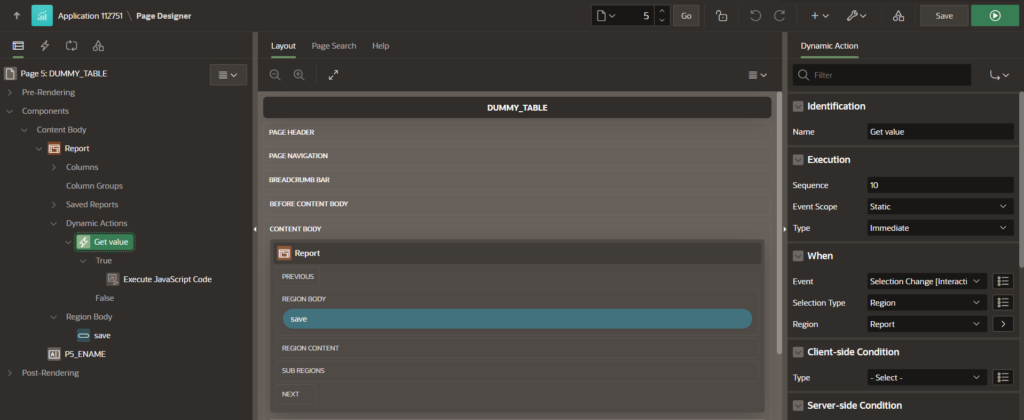
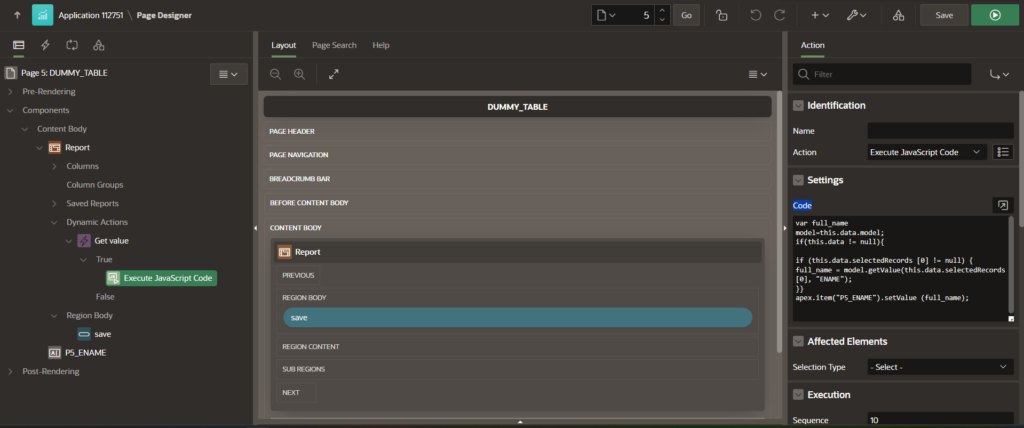
Troubleshooting Tips
If the dynamic action does not work as expected, consider these troubleshooting tips:
- Check for JavaScript Errors:
- Open the browser’s developer tools and inspect the console for errors or warnings, which could indicate syntax issues or incorrect references.
- Ensure the Column Exists:
- Verify that the “ENAME” column is present in your Interactive Grid. If not, update the code to reference the correct column.
- Recreate the Dynamic Action:
- If the dynamic action doesn’t trigger, recreate it with the correct event, selection type, and code.
Conclusion
Retrieving values from the current row in Oracle APEX Interactive Grids can be useful for various scenarios, including setting values in other fields or using them for additional processing. By creating a dynamic action that retrieves and uses the selected value, you can provide a more interactive and dynamic user experience. If you encounter issues, use the troubleshooting tips to resolve common problems.